Change color of each side of cube
Description
Our fragment shader has been assigning the same color to every pixel. Without any form of lighting, this makes it difficult to differentiate the different sides of the cube.
Right now the fragment shader doesn't have a way to determine which pixel belongs to which side, but we can pass it that information from the vertex shader. We can specify output variables from one stage and link them to input variables in another.
We'll define six colors in our vertex shader and use the same color for every six vertices (which define one side of the cube). When OpenGL transforms these vertices into pixels, it will pass along the associated color value to the fragment shader.
Since each vertex for a single side has the same color, every pixel for that side will receive the same color. But we could also assign each vertex a different color and OpenGL will mix the values appropriately to build a color gradient.
Screenshot
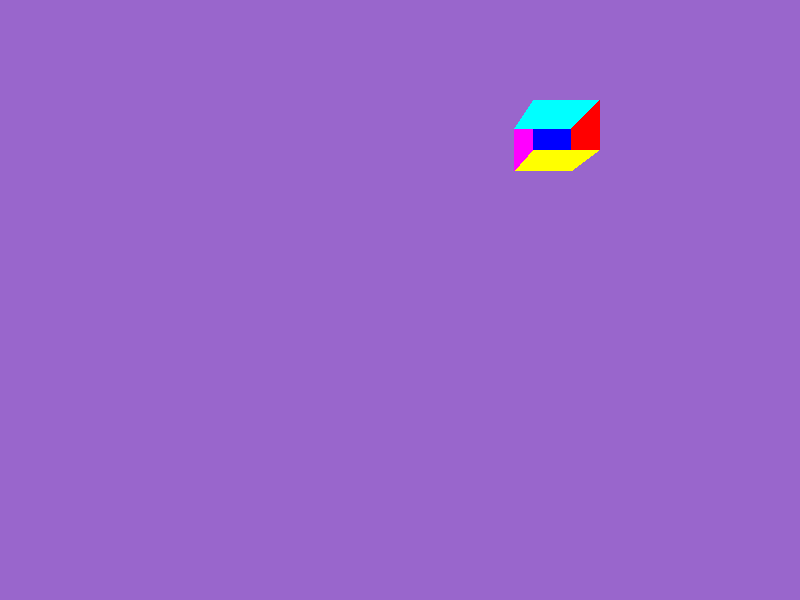
Commands
git clone git@github.com:atsheehan/iridium
cd iridium
git checkout 90ff104e92c5e349ab178fc5734f116fb73b9fe6
cargo run --release
Code Changes
Modified shaders/cube.fragGitHub
@@ -1,7 +1,8 @@11 #version 150
22
3- out vec4 color;
3+ out vec3 color;
4+ in vec3 vertex_color;
45
56 void main() {
6- color = vec4(80.0 / 255.0, 200.0 / 255.0, 120.0 / 255.0, 1.0);
7+ color = vertex_color;
78 }
@@ -1,7 +1,8 @@1 #version 150
2
3- out vec4 color;
4
5 void main() {
6- color = vec4(80.0 / 255.0, 200.0 / 255.0, 120.0 / 255.0, 1.0);
7 }
@@ -1,7 +1,8 @@1 #version 150
2
3+ out vec3 color;
4+ in vec3 vertex_color;
5
6 void main() {
7+ color = vertex_color;
8 }
Modified shaders/cube.vertGitHub
@@ -5,6 +5,8 @@55
66 uniform vec3 position = vec3(2.0, 3.0, 6.0);
77
8+ out vec3 vertex_color;
9+
810 void main() {
911 vec3 near_bottom_left = vec3(0.0, 0.0, 0.0);
1012 vec3 near_bottom_right = vec3(1.0, 0.0, 0.0);
@@ -70,6 +72,14 @@7072 vertices[34] = near_bottom_left;
7173 vertices[35] = far_bottom_left;
7274
75+ vec3 colors[6];
76+ colors[0] = vec3(0.0, 1.0, 0.0);
77+ colors[1] = vec3(1.0, 0.0, 0.0);
78+ colors[2] = vec3(0.0, 0.0, 1.0);
79+ colors[3] = vec3(1.0, 0.0, 1.0);
80+ colors[4] = vec3(0.0, 1.0, 1.0);
81+ colors[5] = vec3(1.0, 1.0, 0.0);
82+
7383 mat4 world_to_clip_transform =
7484 mat4(1.0, 0.0, 0.0, 0.0,
7585 0.0, 1.0, 0.0, 0.0,
@@ -83,4 +93,6 @@8393 0.0, 0.0, 0.0, 1.0);
8494
8595 gl_Position = vec4(vertices[gl_VertexID], 1.0) * model_to_world_transform * world_to_clip_transform;
96+
97+ vertex_color = colors[gl_VertexID / 6];
8698 }
@@ -5,6 +5,8 @@5
6 uniform vec3 position = vec3(2.0, 3.0, 6.0);
7
8 void main() {
9 vec3 near_bottom_left = vec3(0.0, 0.0, 0.0);
10 vec3 near_bottom_right = vec3(1.0, 0.0, 0.0);
@@ -70,6 +72,14 @@70 vertices[34] = near_bottom_left;
71 vertices[35] = far_bottom_left;
72
73 mat4 world_to_clip_transform =
74 mat4(1.0, 0.0, 0.0, 0.0,
75 0.0, 1.0, 0.0, 0.0,
@@ -83,4 +93,6 @@83 0.0, 0.0, 0.0, 1.0);
84
85 gl_Position = vec4(vertices[gl_VertexID], 1.0) * model_to_world_transform * world_to_clip_transform;
86 }
@@ -5,6 +5,8 @@5
6 uniform vec3 position = vec3(2.0, 3.0, 6.0);
7
8+ out vec3 vertex_color;
9+
10 void main() {
11 vec3 near_bottom_left = vec3(0.0, 0.0, 0.0);
12 vec3 near_bottom_right = vec3(1.0, 0.0, 0.0);
@@ -70,6 +72,14 @@72 vertices[34] = near_bottom_left;
73 vertices[35] = far_bottom_left;
74
75+ vec3 colors[6];
76+ colors[0] = vec3(0.0, 1.0, 0.0);
77+ colors[1] = vec3(1.0, 0.0, 0.0);
78+ colors[2] = vec3(0.0, 0.0, 1.0);
79+ colors[3] = vec3(1.0, 0.0, 1.0);
80+ colors[4] = vec3(0.0, 1.0, 1.0);
81+ colors[5] = vec3(1.0, 1.0, 0.0);
82+
83 mat4 world_to_clip_transform =
84 mat4(1.0, 0.0, 0.0, 0.0,
85 0.0, 1.0, 0.0, 0.0,
@@ -83,4 +93,6 @@93 0.0, 0.0, 0.0, 1.0);
94
95 gl_Position = vec4(vertices[gl_VertexID], 1.0) * model_to_world_transform * world_to_clip_transform;
96+
97+ vertex_color = colors[gl_VertexID / 6];
98 }