Move cube around via affine transformation
Description
Our coordinates changed from (-1.0, 1.0) to (0.0, 8.0), but our cube is still sitting at (0.0, 0.0, 0.0) in the bottom-left corner of the screen. We hard-coded the cube's vertex positions between (0.0, 0.0, 0.0) and (1.0, 1.0, 1.0).
We could update the cube's vertices to the position we want them in, but we can also move the cube around via another matrix transformation.
We'll keep our cube vertices positioned at the origin and call this coordinate system the model space. We want to move this cube to another location in our world space.
We can use a 4x4 translation matrix to move a position by a given amount.
With this approach, we can reuse the same vertices to draw many cubes in different positions just by varying the transformation matrix. For a handful of vertices this may not be that important, but for models with thousands of vertices this could have significant performance savings.
Screenshot
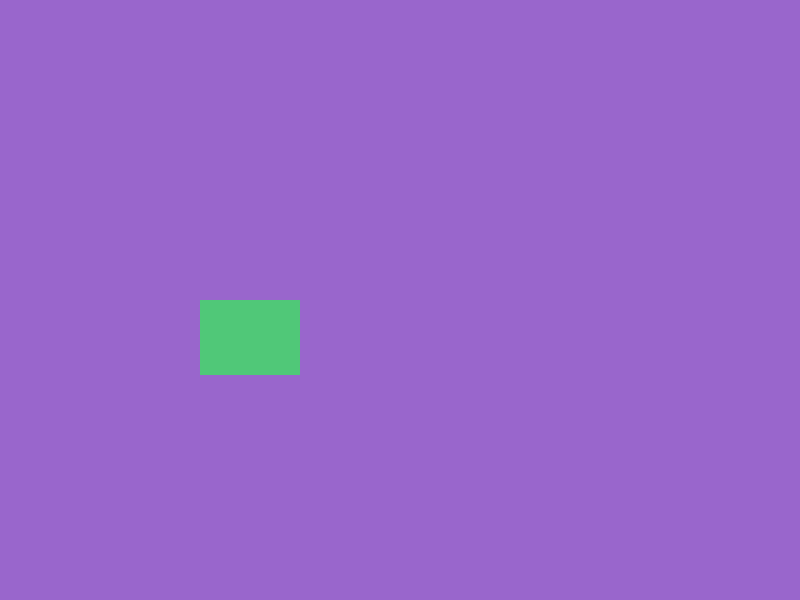
Commands
git clone git@github.com:atsheehan/iridium
cd iridium
git checkout b01fb7ac07531bba1e3888baa1ef512c786834fb
cargo run --release
Code Changes
Modified shaders/cube.vertGitHub
@@ -10,6 +10,8 @@1010 const float HEIGHT = TOP - BOTTOM;
1111 const float DEPTH = FAR - NEAR;
1212
13+ uniform vec3 position = vec3(2.0, 3.0, 6.0);
14+
1315 void main() {
1416 vec3 near_bottom_left = vec3(0.0, 0.0, 0.0);
1517 vec3 near_bottom_right = vec3(1.0, 0.0, 0.0);
@@ -81,5 +83,11 @@8183 0.0, 0.0, 2.0 / DEPTH, -(FAR + NEAR) / DEPTH,
8284 0.0, 0.0, 0.0, 1.0);
8385
84- gl_Position = vec4(vertices[gl_VertexID], 1.0) * world_to_clip_transform;
86+ mat4 model_to_world_transform =
87+ mat4(1.0, 0.0, 0.0, position.x,
88+ 0.0, 1.0, 0.0, position.y,
89+ 0.0, 0.0, 1.0, position.z,
90+ 0.0, 0.0, 0.0, 1.0);
91+
92+ gl_Position = vec4(vertices[gl_VertexID], 1.0) * model_to_world_transform * world_to_clip_transform;
8593 }
@@ -10,6 +10,8 @@10 const float HEIGHT = TOP - BOTTOM;
11 const float DEPTH = FAR - NEAR;
12
13 void main() {
14 vec3 near_bottom_left = vec3(0.0, 0.0, 0.0);
15 vec3 near_bottom_right = vec3(1.0, 0.0, 0.0);
@@ -81,5 +83,11 @@81 0.0, 0.0, 2.0 / DEPTH, -(FAR + NEAR) / DEPTH,
82 0.0, 0.0, 0.0, 1.0);
83
84- gl_Position = vec4(vertices[gl_VertexID], 1.0) * world_to_clip_transform;
85 }
@@ -10,6 +10,8 @@10 const float HEIGHT = TOP - BOTTOM;
11 const float DEPTH = FAR - NEAR;
12
13+ uniform vec3 position = vec3(2.0, 3.0, 6.0);
14+
15 void main() {
16 vec3 near_bottom_left = vec3(0.0, 0.0, 0.0);
17 vec3 near_bottom_right = vec3(1.0, 0.0, 0.0);
@@ -81,5 +83,11 @@83 0.0, 0.0, 2.0 / DEPTH, -(FAR + NEAR) / DEPTH,
84 0.0, 0.0, 0.0, 1.0);
85
86+ mat4 model_to_world_transform =
87+ mat4(1.0, 0.0, 0.0, position.x,
88+ 0.0, 1.0, 0.0, position.y,
89+ 0.0, 0.0, 1.0, position.z,
90+ 0.0, 0.0, 0.0, 1.0);
91+
92+ gl_Position = vec4(vertices[gl_VertexID], 1.0) * model_to_world_transform * world_to_clip_transform;
93 }